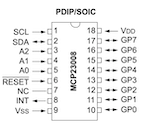 |
PU2CLR MCP23008 Arduino Library
1.0.7
Arduino Library for MCP23008 Device - By Ricardo Lima Caratti
|
Go to the documentation of this file.
24 #define REG_IODIR 0x00
26 #define REG_GPINTEN 0x02
27 #define REG_DEFVAL 0x03
28 #define REG_INTCON 0x04
29 #define REG_IOCON 0x05
32 #define REG_INTCAP 0x08
36 #define GPIO_INPUT 0xFF
37 #define GPIO_OUTPUT 0x00
39 #define INTERRUPT_ODR_OPEN_DRAIN 1
40 #define INTERRUPT_ODR_ACTIVE_DRIVE 0
41 #define INTERRUPT_INTPOL_ACTIVE_HIGH 1
42 #define INTERRUPT_INTPOL_ACTIVE_LOW 0
53 #define CHECK_BIT_HIGH(x,y) ( x & (1
<< y) )
88 void setup(uint8_t i2c = 0x20, uint8_t io =
GPIO_OUTPUT,
int reset_pint = -1,
long i2c_freq = 100000);
95 void setIoCon(uint8_t INTPOL, uint8_t ODR, uint8_t HAEN, uint8_t DISSLW, uint8_t SEQOP);
98 void setInterrupt(uint8_t polatity = 0, uint8_t openDrainOutput = 0);
101 void gpioWrite(uint8_t gpio, uint8_t value);
113 this->gpios = getRegister(
REG_GPIO);
160 return (byteValue & (1 << bitNumber)) != 0;
uint8_t getINTCAP()
Returns the last value of INTCAP register (value immediately after the last interrupt event)
Definition: pu2clr_mcp23008.h:133
#define REG_INTCAP
The INTCAP register captures the GPIO port value at the time the interrupt occurred.
Definition: pu2clr_mcp23008.h:32
uint8_t intcap
Definition: pu2clr_mcp23008.h:81
uint8_t getRegister(uint8_t reg)
Gets the corrent register information.
Definition: pu2clr_mcp23008.cpp:97
#define REG_DEFVAL
The default comparison value is configured in the DEFVAL register.
Definition: pu2clr_mcp23008.h:27
void turnGpioOn(uint8_t gpio)
Turns a given GPIO port on (high level)
Definition: pu2clr_mcp23008.cpp:128
uint8_t getGPIOS()
Returns the current MCP GPIO pin levels.
Definition: pu2clr_mcp23008.h:111
bool gpioRead(uint8_t gpio)
Reads the status (high or low) of a given GPIO.
Definition: pu2clr_mcp23008.cpp:163
int reset_pin
Digital Arduino pin to control the MCP2300 RESET.
Definition: pu2clr_mcp23008.h:83
void invertGpioPolarity()
Inverts the polarity of the all GPIO port bits.
Definition: pu2clr_mcp23008.cpp:300
Definition: pu2clr_mcp23008.h:75
void setup(uint8_t i2c=0x20, uint8_t io=GPIO_OUTPUT, int reset_pint=-1, long i2c_freq=100000)
Starts the MCP23008.
Definition: pu2clr_mcp23008.cpp:76
void setInterrupt(uint8_t polatity=0, uint8_t openDrainOutput=0)
Configures the MCP23008 interrupt feature.
Definition: pu2clr_mcp23008.cpp:316
#define GPIO_OUTPUT
Definition: pu2clr_mcp23008.h:37
uint8_t intf
Definition: pu2clr_mcp23008.h:82
#define REG_GPIO
The GPIO register reflects the value on the port.
Definition: pu2clr_mcp23008.h:33
bool isBitValueHigh(uint8_t byteValue, uint8_t bitNumber)
Checks if the Bit Value of a given bit position is high.
Definition: pu2clr_mcp23008.h:159
#define REG_IOCON
The IOCON register contains several bits for configuring the device. See method: setIoCon.
Definition: pu2clr_mcp23008.h:29
void gpioWrite(uint8_t gpio, uint8_t value)
Sets a given value (high(1) or low(0) ) to a given gpio pin.
Definition: pu2clr_mcp23008.cpp:175
void pullUpGpioOn(uint8_t gpio)
Turns intenal pull up resistor ON to a given GPIO PIN (high level)
Definition: pu2clr_mcp23008.cpp:218
void setRegister(uint8_t reg, uint8_t value)
Sets a value to a given register.
Definition: pu2clr_mcp23008.cpp:113
void reset()
Resets the MCP23008.
Definition: pu2clr_mcp23008.cpp:54
void registerDigitalWrite(uint8_t mcp_register, uint8_t bit_position, uint8_t value)
Sets High or Low to a given position in a given MCP23008 register.
Definition: pu2clr_mcp23008.cpp:204
uint8_t gpios
REG_GPIO shadow register.
Definition: pu2clr_mcp23008.h:80
void setIoCon(uint8_t INTPOL, uint8_t ODR, uint8_t HAEN, uint8_t DISSLW, uint8_t SEQOP)
Sets the IO Configurarion gerister.
Definition: pu2clr_mcp23008.cpp:264
mcp23008_ioncon getIoCon()
Returns the IOCON content.
Definition: pu2clr_mcp23008.cpp:284
#define REG_INTF
The INTF register reflects the interrupt condition on the port pins of any pin that is enabled for in...
Definition: pu2clr_mcp23008.h:31
uint8_t lookForDevice()
Look for MCP23008 device I2C Address.
Definition: pu2clr_mcp23008.cpp:33
void pullUpGpioOff(uint8_t gpio)
Turns intenal pull up resistor OFF to a given GPIO PIN (low level)
Definition: pu2clr_mcp23008.cpp:236
#define REG_IPOL
The IPOL register allows the user to configure the polarity on the corresponding GPIO port bits.
Definition: pu2clr_mcp23008.h:25
#define REG_GPPU
The GPPU register controls the pull-up resistors for the port pins.
Definition: pu2clr_mcp23008.h:30
void interruptGpioOn(uint8_t gpio, uint8_t bitCompare=1)
Sets the interrupt-on-change feature to a given GPIO pin.
Definition: pu2clr_mcp23008.cpp:336
uint8_t raw
Definition: pu2clr_mcp23008.h:72
uint8_t i2cAddress
Default i2c address.
Definition: pu2clr_mcp23008.h:79
void setGPIOS(uint8_t value)
Sets a value to the GPIO Register.
Definition: pu2clr_mcp23008.h:123
bool registerDigitalRead(uint8_t mcp_register, uint8_t bit_position)
Reads the status (high or low) of a given bit (position) of a given MCP23008 register.
Definition: pu2clr_mcp23008.cpp:189
#define REG_IODIR
Controls the direction of the data I/O. When a bit is set, the corresponding pin becomes an input....
Definition: pu2clr_mcp23008.h:24
uint8_t getINTF()
Returns the last value of INTF register.
Definition: pu2clr_mcp23008.h:145
#define REG_GPINTEN
The GPINTEN register controls the interrupt-on-change feature for each pin.
Definition: pu2clr_mcp23008.h:26
void setClock(long freq)
Sets I2C bus to a given frequency.
Definition: pu2clr_mcp23008.h:169
void turnGpioOff(uint8_t gpio)
Turns a given GPIO port off (low level)
Definition: pu2clr_mcp23008.cpp:146